Cross-fading is linear by default, meaning it moves the AnimancerNode.Weight
towards the AnimancerNode.TargetWeight
at a constant AnimancerNode.FadeSpeed
calculated when starting the fade. But that doesn't always give the smoothest transitions so Animancer allows you to modify a fade progress using a standard Easing.Function
or any custom delegate.
The 3D Game Kit sample demonstrates the use of this feature to smooth out the transitions between its Idle animations.
To use the system, simply play something to start a fade like you normally would.
void EasingExample(AnimancerComponent animancer, AnimationClip clip)
{
AnimancerState state = animancer.Play(clip, 0.25f);
Then call any of the FadeGroupExtensions.SetEasing
methods.
// Assign any function that takes a float and returns a float.
state.FadeGroup.SetEasing(t => t * t);// Square the 0-1 value to start slow and end fast.
// The Easing class has lots of standard mathematical curve functions.
state.FadeGroup.SetEasing(Easing.Sine.InOut);
// Or you can use the Easing.Function enum.
state.FadeGroup.SetEasing(Easing.Function.SineInOut);
}
Animation Curves
If you want to manually edit the fade curve in the Inspector, you can use an AnimationCurve
and assign its Evaluate
method as the easing function.
[SerializeField] private AnimationCurve _FadeCurve;
void EasingExample(AnimancerComponent animancer, AnimationClip clip)
{
AnimancerState state = animancer.Play(clip, 0.25f);
state.FadeGroup.SetEasing(_FadeCurve.Evaluate);
}
If you want a library of standard curves to choose from, you can download the Animancer Curve Presets from here and use them as a starting point for your own curves. Note that these curves were configured by hand with the minimum number of keyframes for efficiency. They don't cover the exact same set of functions as the Easing
class and any that have the same name will have slight differences in their exact values.
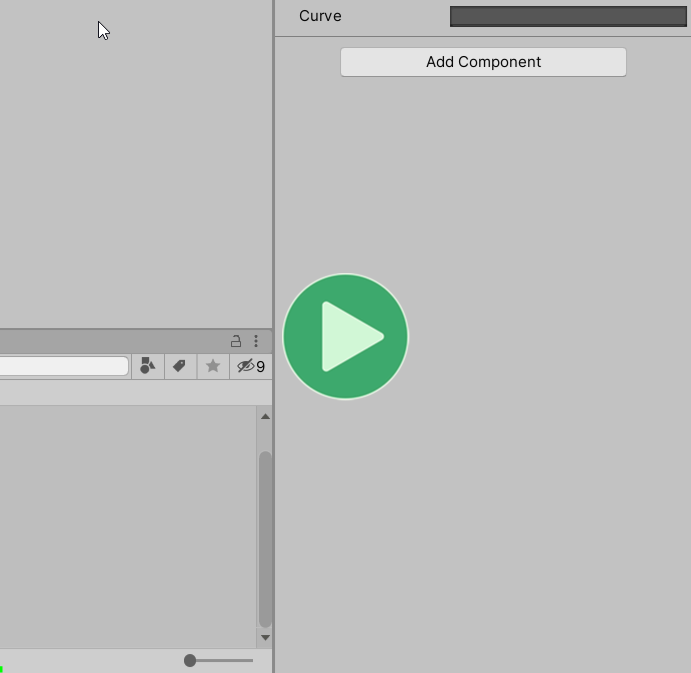