After you Download Animancer, you can add an AnimancerComponent
to your model and control it with a script:
- Don't create an Animator Controller like you normally would. Animancer doesn't need them.
- Add a reference to the
AnimancerComponent
(let’s call itanimancer
). - Add a reference to the
AnimationClip
asset you want to play (let’s call itclip
). - Then you simply call
animancer.Play(clip)
.
AnimancerComponent.Play
returns an AnimancerState
that can be used to access and control the animation details such as Speed
and Time
.
Sample
The Quick Play sample gives a detailed step by step explanation of how to play animations, but here's an overview:
using Animancer;
using UnityEngine;
public class PlayAnimation : MonoBehaviour
{
[SerializeField] private AnimancerComponent _Animancer;
[SerializeField] private AnimationClip _Clip;
protected virtual void OnEnable()
{
_Animancer.Play(_Clip);
// You can manipulate the animation using the returned AnimancerState:
AnimancerState state = _Animancer.Play(_Clip);
state.Speed = ... // See the Fine Control samples.
state.Time = ... // See the Fine Control samples.
state.NormalizedTime = ... // See the Fine Control samples.
state.Events(this).OnEnd = ... // See End Events.
// If the animation was already playing, it will continue from the current time.
// So to force it to play from the beginning you can just reset the Time:
_Animancer.Play(_Clip).Time = 0;
}
}
Simply attach that script to an object and assign its references in the Inspector:
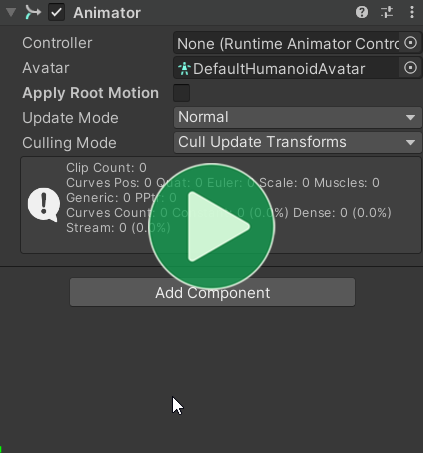
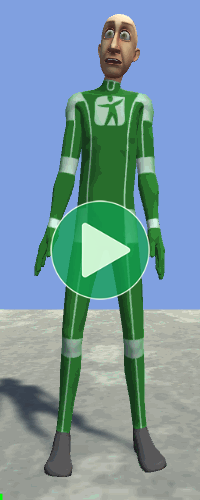
Play Methods
The AnimancerComponent
class has several Play
methods with different parameter sets depending on what you want to do.
Play Immediately
Play(AnimationClip clip)
Play(AnimancerState state)
These methods immediately snap the character into the new animation. This is particularly good for Sprite
animations such as those in the Directional Basics sample because there is nothing to blend, the character can only show one Sprite
at a time.
The differences between these parameters are explained on the States page.
Cross Fade
Play(AnimationClip clip, float fadeDuration, FadeMode mode = FadeMode.FixedSpeed)
Play(AnimancerState state, float fadeDuration, FadeMode mode = FadeMode.FixedSpeed)
These methods fade the new animation in over time and fade the old animation out at the same time, which is known as Cross Fading. This is often much better for Skeletal Animations because it allows a character model to smoothly transition from the ending pose of one animation into the starting pose of another animation without requiring both poses to be exactly the same. It also means that the transition can still be smooth if an animation is interrupted at any time. The Transitions sample demonstrates the differences between playing animations immediately and cross fading between them.
Note that the FadeMode
parameter is optional so the following two lines will both do the same thing:
animancer.Play(clip, 0.25f)
animancer.Play(clip, 0.25f, FadeMode.FixedSpeed)
Transition
Play(ITransition transition)
Play(ITransition transition, float fadeDuration, FadeMode mode = FadeMode.FixedSpeed)
These methods allow Transitions to apply all their details in a single call. This includes the fadeDuration
(unless you use the second method to specify it yourself) as well as any other details defined in the transition
such as Speed, Start Time, and any Animancer Events which can all be configured in the Inspector when using the inbuilt transition types instead of hard coding those details into your script.
The Transitions sample demonstrates how to use these methods.
Try Play
TryPlay(object key)
TryPlay(object key, float fadeDuration, FadeMode mode = FadeMode.FixedSpeed)
Unlike the above methods, these ones use the key
to find an existing State to play but can't create new ones if they aren't yet registered (trying to do so will return null
). Once the state is found, they simply call the corresponding Play Immediately or Cross Fade method.
The Named Character sample demonstrates how to use these methods.
Play State
AnimancerState state;
state.Play();
state.StartFade(1, 0.25f);
Unlike the methods in AnimancerComponent
and AnimancerLayer
, calling methods on a state will only affect that state and won't stop any others that are playing. These methods aren't useful in most circumstances, but they are available if you need more complex control over things.
Pausing
There are several different ways you can pause and stop individual animations or all of them at once:
using Animancer;
using UnityEngine;
public class PausingExample : MonoBehaviour
{
[SerializeField] private AnimancerComponent _Animancer;
[SerializeField] private AnimationClip _Clip;
// Freeze a single animation on its current frame:
public void PauseClip()
{
AnimancerState state = _Animancer.States[_Clip];
state.IsPlaying = false;
}
// Freeze all animations on their current frame:
public void PauseAll()
{
_Animancer.Graph.PauseGraph();
}
// Stop a single animation from affecting the character and rewind it to the start:
public void StopClip()
{
_Animancer.Stop(_Clip);
// Or you can call it on the state directly:
AnimancerState state = _Animancer.States[_Clip];
state.Stop();
}
// Stop all animations from affecting the character and rewind them to the start:
public void StopAll()
{
_Animancer.Stop();
}
// Stop all previous animations and play a new one:
public void Play()
{
_Animancer.Play(_Clip);
}
// Play an animation without affecting any others:
public void PlayIsolatedClip()
{
AnimancerState state = _Animancer.States.GetOrCreate(_Clip);
state.Play();
}
}
States | The most common type of AnimancerState is a ClipState which plays a single AnimationClip and is registered in an internal dictionary using that clip as the key. |
Keys | Animancer keeps track of states in an internal dictionary which you can use to look them up via their keys. |
Inspector | Animancer shows the real-time details of all animations in the Inspector so you can observe what they are doing and control them manually for testing and debugging purposes. |
Component Types | There are several different kinds of AnimancerComponent and you can make your own to add or modify functionality. |
Playing in Edit Mode | Playing animations in Edit Mode is very easy. |
Directional Animation Sets | Group animations into sets of up/right/down/left (including or excluding diagonals) to implement characters who can face any direction while performing various actions. |