The Playables API which Animancer is built around works using a tree-like structure of nodes known as a PlayableGraph
. The following diagram shows how Animancer arranges the graph connections:
- The root
AnimancerGraph
can have multipleAnimancerLayer
s connected to it. - Each
AnimancerLayer
can have multipleAnimancerState
s connected to it. MixerState
s are a type ofAnimancerState
which can have other states connected to them, including other mixers.- Most of the time a new state is connected to its parent when created, but if you need to you can use
AnimancerState.SetParent
to reconfigure the graph dynamically.
Playable Graph Visualizer
The Playable Graph Visualizer is a tool made by Unity for displaying PlayableGraph
s. If you have it in your project, the Live Inspector context menu will include a function to open the visualizer and set it to show the current graph.
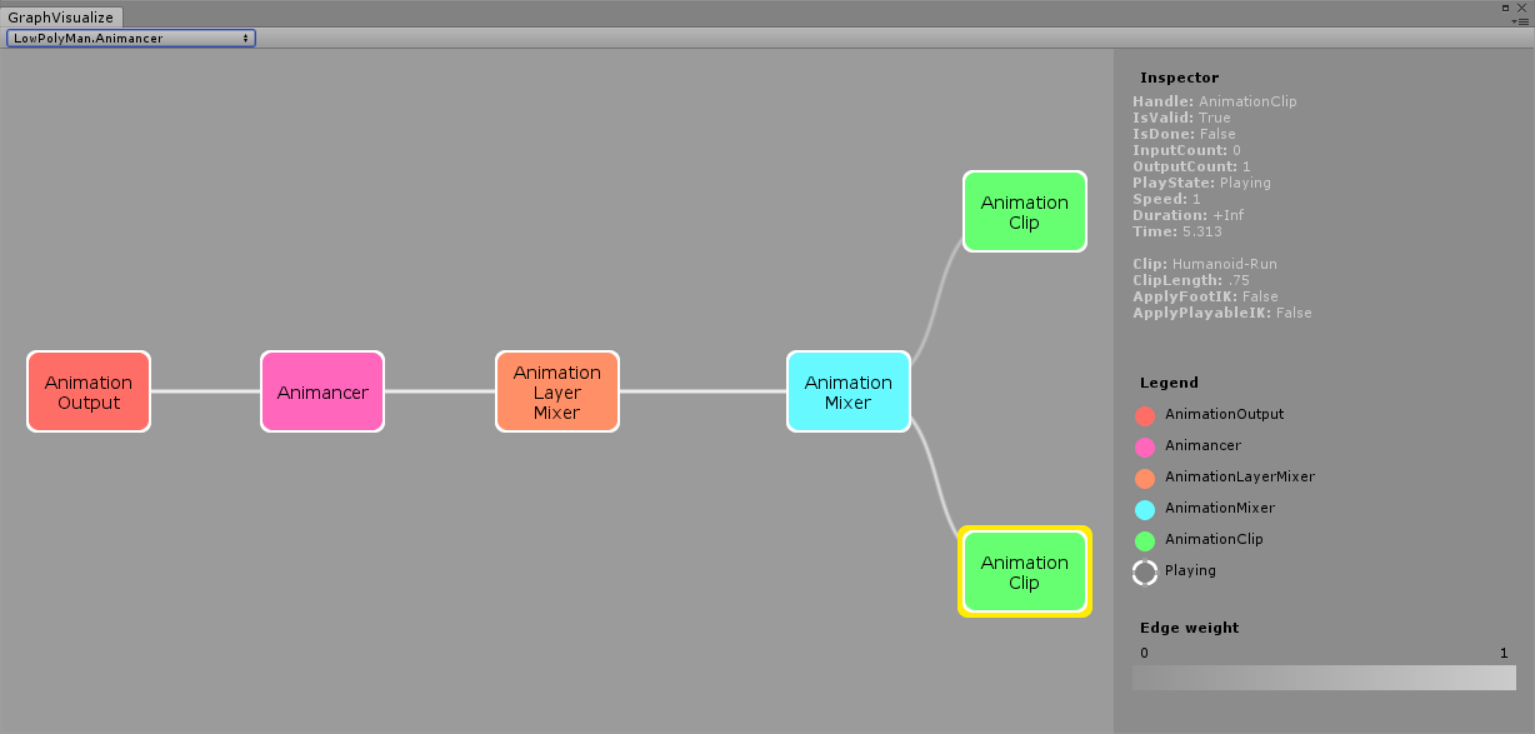
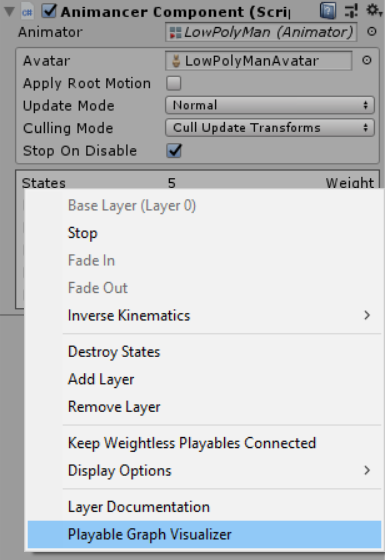
Iterating
All of the core classes in Animancer implement IEnumerable<AnimancerState>
, meaning that you can use them in foreach
loops to iterate through their child states:
private void LogAllStates(AnimancerComponent animancer)
{
Debug.Log("Logging all states in " + animancer);
foreach (AnimancerState state in animancer)
{
Debug.Log("Found state " + state);
LogAllStates(state, 1);
}
}
private void LogAllStates(AnimancerState state, int indent = 0)
{
foreach (AnimancerState childState in state)
{
string message = "Found child state " + state;
for (int i = 0; i < indent; i++)
message = " " + message;
Debug.Log(message);
LogAllStates(childState, indent + 1);
}
}