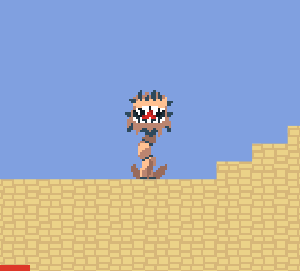
The basic IdleState
used by the Maw Flower enemy is really simple.
It just has a Serialized Field for an animation which it plays when the state is entered:
public class IdleState : CharacterState
{
[SerializeField] private ClipTransition _Animation;
public override void OnEnterState()
{
base.OnEnterState();
Character.Animancer.Play(_Animation);
}
Since this will be the character's starting state, it also has an OnValidate
method to apply the currently assigned animation's first frame so that the character in the scene automatically shows the first Sprite
of their Idle animation:
#if UNITY_EDITOR
protected override void OnValidate()
{
base.OnValidate();
_Animation?.Clip.EditModeSampleAnimation(Character);
}
#endif
}
The base CharacterState
has a MovementSpeedMultiplier
property which states can override
to allow movement, but since IdleState
doesn't it will always return 0
:
public virtual float MovementSpeedMultiplier => 0;